Certain use cases may require your organization to change an end-user's consent profile outside the Sourcepoint first layer and privacy manager messages. The postCustomConsent
command can be used to edit an end-user's consent profile by changing the opt-in status for consented vendors, categories (purposes), and legitimate interest categories (purposes).
Use Case
Apply vendor-specific consent experiences to handle social media plugins. Or apply consent because an end-user accepts the website's T&Cs or a contract provided by a publisher or advertiser.
Command
window._sp_.gdpr.postCustomConsent((vendorConsents, success) => console.log(vendorConsents, success), [vendorIds], [purposeIds], [legitimateInterestPurposeIds] );
Parameter | Type | Description |
---|---|---|
vendorIds | string | List of vendor IDs that will be consented to by end-user. Comma delimited. |
purposeIds | string | List of purpose IDs who use consent as a legal basis that will be consented to by end-user. Comma delimited. |
legitimateInterestPurposeIds | string | List of purpose IDs who use legitimate interest as a legal basis that will be opted-into by end-user. Comma delimited. |
You will need to consent to both vendors and purposes. Consenting to a vendor only does not include the purposes.
Implement postCustomConsent
postCustomConsent
In order to implement the postCustomConsent
command on your website property, you will need to:
- Implement a button or link on your website property with an element ID. The suggested element ID is "postCustomConsent".
- Create a function that executes the
postCustomConsent
command with purposes and vendors that will be consented to when triggered. - Use the
document.getElementbyId
method to return the button or link ID and call your function for anonclick
event.
<!--Create modal with a button-->
<div id="myModal" class="modal">
<div class="modal-content">
<p>Give consent to X, Y, Z vendors and purposes.</p>
<button id="postCustomConsent">Give consent to X Y Z</button>
</div>
</div>
<script>
//calls function when button is clicked
document.getElementById("postCustomConsent").onclick = function() {myFunction()};
//function that executes postCustomConsent command
function myFunction() {
window._sp_.gdpr.postCustomConsent((vendorConsents, success) => console.log(vendorConsents, "postCustomConsent was successful: " + success), [vendorIds], [purposeIds], [legitimateInterestPurposeIds] );
}
</script>
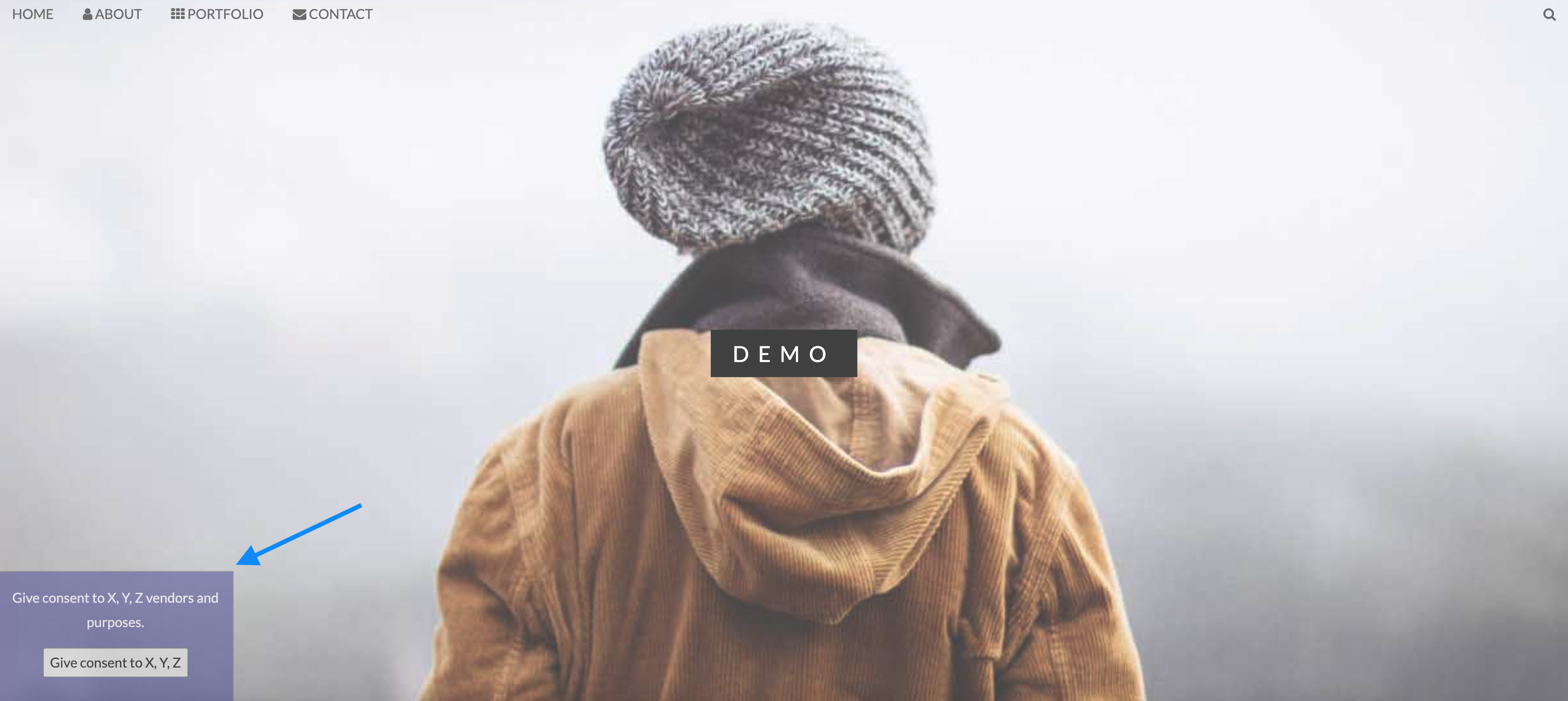
Response
{
"categories": [
"abcde01e32bd4d27654ba1d5"
],
"consentStatus": {
"consentedAll": true,
"consentedToAny": true,
"granularStatus": {
"defaultConsent": true,
"previousOptInAll": true,
"purposeConsent": "SOME",
"purposeLegInt": "ALL",
"vendorConsent": "SOME",
"vendorLegInt": "SOME"
},
"hasConsentData": true,
"rejectedAny": true,
"rejectedLI": true
},
"consentUUID": "d6f6c2e8-4af8-4156-8f8d-1bfbf1d8c591_20",
"cookies": [
{
"key": "consentUUID",
"maxAge": 31536000,
"session": false,
"shareRootDomain": false,
"value": "d6f6c2e8-4af8-4156-8f8d-1bfbf1d8c591_20"
}
],
"customVendorsResponse": {
"consentedPurposes": [
{
"name": "Select basic ads",
"_id": "5fd7e01e32bd4d27654ba1d5"
}
],
"consentedVendors": [
{
"name": "Vendor name",
"vendorType": "CUSTOM",
"_id": "5f1b2fbeb8e05c306f2a1ed6"
}
],
"legIntPurposes": [
{
"name": "Create a personalised content profile",
"_id": "5fd7e01e32bd4d27654ba1ed"
}
]
},
"grants": {
"vendorId": {
"purposeGrants": {
"purposeId": false
},
"vendorGrant": false
}
},
"legIntCategories": [
"5fd7e01e32bd4d27654ba1ed"
],
"legIntVendors": [
"5f1b2fbeb8e05c306f2a1ed6"
],
"rejectedAny": true,
"specialFeatures": [],
"vendors": [
"5f1b2fbeb8e05c306f2a1ed6"
]
}
postCustomConsent was successful: true
schema:
type: object
description: Returned response for successful postCustomConsent request
properties:
categories:
type: array
description: Purposes consented to by end-user
items:
type: string
description: Purpose ID
consentStatus:
type: object
description: Consent statuses for the end-user
properties:
consentedAll:
type: boolean
description: Is end-user consented to all purposes and vendors?
consentedToAny:
type: boolean
description: Is end-user consented to any purposes or vendors?
granularStatus:
type: object
properties:
defaultConsent:
type: boolean
description:
previousOptInAll:
type: boolean
description: End-user previously opted into for all vendors and purposes.
purposeConsent:
type: string
description: Purposes consented to by end-user. ALL | SOME | NONE
purposeLegInt:
type: string
description: Purposes who use legitimate interest as a legal basis. ALL | SOME | NONE
vendorConsent:
type: string
description: Vendors who was consented to by end-user. ALL | SOME | NONE
vendorLegInt:
type: string
description: Vendors who use legitimate interest as a legal basis for a purpose and was consented to by end-user. ALL | SOME | NONE
hasConsentData:
type: boolean
description: Does end-user have consent data?
rejectedAny:
type: boolean
description: Did end-user reject any purposes or vendors?
rejectedLI:
type: boolean
description: Did end-user reject any purposes that use legitimate interest as a legal basis?
consentUUID:
type: string
description: consent UUID
cookies:
type: array
description: Cookies create/updated
items:
type: object
properties:
key:
type: string
description: Name of cookie
maxAge:
type: number
description: Cookie duration
session:
type: boolean
description: Is the cookie a session cookie?
shareRootDomain:
type: boolean
description: Is the cookie written to the root domain?
value:
type: string
description: Value of the cookie
customVendorsResponse:
type: object
description: Purposes and vendors consented to by end-user
properties:
consentedPurposes:
type: array
description: List purposes consented to by end-user
items:
type: object
properties:
name:
type: string
description: Name of the purpose
_id:
type: string
description: Purpose ID
consentedVendors:
type: array
description: Vendors consented to by the end-user
items:
type: object
properties:
name:
type: string
description: Name of the vendor
vendorType:
type: string
description: Vendor type
_id:
type: string
description: Vendor ID
legIntPurposes:
type: array
description: Purposes who use legitimate interest as a legal basis accepted by the end-user
items:
type: object
properties:
name:
type: string
description: Name of the purpose
_id:
type: string
description: Purpose ID
grants:
type: object
description: Applicable purposes that were consented to for each vendor
properties:
vendorId: #value will be vendor's ID
type: object
properties:
purposeGrants:
type: object
description: Applicable purposes for a vendor where consent was given/not given
properties:
purposeId: #value will be purpose's ID
type: boolean
description: Is consent given for the purpose?
vendorGrant:
type: boolean
description: true if consent is given for all purposes for a vendor | false if consent for one or more purposes are not given to the vendor.
legIntCategories:
type: array
description: Purpose who use legitimate interest as a legal basis and was consented to by end-user
items:
type: string
description: Purpose ID
legIntVendors:
type: array
description: Vendors who use legitimate interest as a legal basis for a purpose and was consented to by end-user
items:
type: string
description: Vendor ID
rejectedAny:
type: boolean
description: Did end-user reject any purposes or vendors?
specialFeatures:
type: array
description: IAB special features. Not applicable to GDPR Standard
items:
type: string
description: Special feature. Not applicable to GDPR Standard
vendors:
type: array
description: Vendors consented to by end-user
items:
type: string
description: Vendor ID